In today’s digital world, including a star rating system in PHP and MySQL is critical for gathering user input and increasing engagement. This detailed article will follow you through the process of building a 5-star rating system using PHP, MySQL, and even jQuery for extra interactivity.
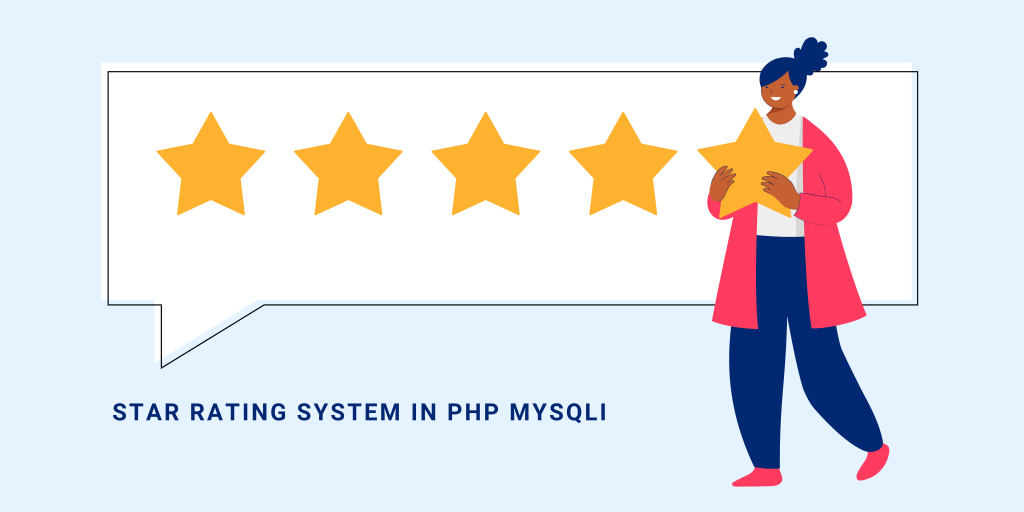
- Understanding the Importance of a Star Rating System in Php
- Setting Up Your Development Environment
- Creating the Database Structure
- Building the Star Rating Form
- Handling User Input with PHP
- Storing Ratings in the Database
- Displaying Average Ratings
- Enhancing the User Experience with AJAX
- Securing Your Star Rating System in Php
- Testing and Deployment
Understanding The Importance Of A Star Rating System in Php
Before getting into the technical details, it’s critical to understand the importance of a star rating system in PHP and MySQL. Whether you run an e-commerce platform, a review site, or another type of online business, a 5-star rating system offers customers vital data and builds trust in your company.
Setting Up Your Development Environment
To start developing your star rating system, make sure you have a local development environment set up with PHP, MySQL, and jQuery, if necessary. Tools like XAMPP or WAMP can help with this setup, letting you focus on the development chores ahead.
Creating The Database Structure
A solid foundation begins with a well-designed database structure. In MySQL, create tables for storing user information, item details, and ratings. Create associations between these tables to ensure data integrity and efficient querying.
-- MySQL Table Creation
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL
);
CREATE TABLE items (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL
);
CREATE TABLE ratings (
id INT AUTO_INCREMENT PRIMARY KEY,
user_id INT,
item_id INT,
rating INT,
FOREIGN KEY (user_id) REFERENCES users(id),
FOREIGN KEY (item_id) REFERENCES items(id)
);
Building the Star Rating Form
Create a user-friendly star rating form with HTML, CSS, and perhaps some jQuery for interactive features. Ensure that the form has clear instructions and an intuitive design so that people can easily submit ratings.
<!-- HTML Rating Form -->
<form action="submit_rating.php" method="post">
<input type="hidden" name="item_id" value="1">
<div class="stars">
<!-- Stars generated dynamically using jQuery -->
</div>
<button type="submit">Submit Rating</button>
</form>
Handling User Input with PHP
Use PHP scripts to process form submissions securely. Validate and sanitize user input to protect against common vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks.
// PHP Handling User Input
$item_id = $_POST['item_id'];
$user_id = $_SESSION['user_id'];
$rating = $_POST['rating'];
// Validate and sanitize input before processing
Storing Ratings In The Database
After obtaining user ratings, securely store them in the MySQL database. To avoid SQL injection and ensure data integrity, use prepared statements or parameterized queries.
// PHP Storing Ratings in Database
// Assuming you've established a database connection
$sql = "INSERT INTO ratings (user_id, item_id, rating) VALUES (?, ?, ?)";
$stmt = $pdo->prepare($sql);
$stmt->execute([$user_id, $item_id, $rating]);
Displaying Average Ratings
Calculate and show the average rating for each item using the stored data. Use SQL queries to aggregate ratings and display them in a visually pleasing manner.
// PHP Displaying Average Ratings
$item_id = 1; // Example item ID
$sql = "SELECT AVG(rating) AS average_rating FROM ratings WHERE item_id = ?";
$stmt = $pdo->prepare($sql);
$stmt->execute([$item_id]);
$result = $stmt->fetch(PDO::FETCH_ASSOC);
$average_rating = $result['average_rating'];
echo "Average Rating: " . number_format($average_rating, 1);
Enhancing The User Experience With Ajax
Use AJAX capabilities to give real-time changes without requiring page reloads. Allow users to submit ratings and display average ratings dynamically, which would improve the overall user experience.
// jQuery AJAX Example
$.ajax({
url: 'submit_rating.php',
type: 'post',
data: formData,
success: function(response) {
// Handle success response
},
error: function(xhr, status, error) {
// Handle error response
}
});
Securing Your Star Rating System
Implement strong security measures like as input validation, data sanitization, and session management to ensure your star rating system’s integrity. Regularly update your system to solve security flaws and safeguard user data.
Testing and Deployment
Test your star rating system extensively on many browsers and devices to confirm compatibility and performance. Once testing is completed, move your system to production and check its performance on a regular basis.
Conclusion
By following this complete guide, you can build a strong 5-star rating system in PHP and MySQL that increases user engagement and gives vital feedback for your online platform. Begin developing your star rating system now and enable your users to make educated judgments with ease.