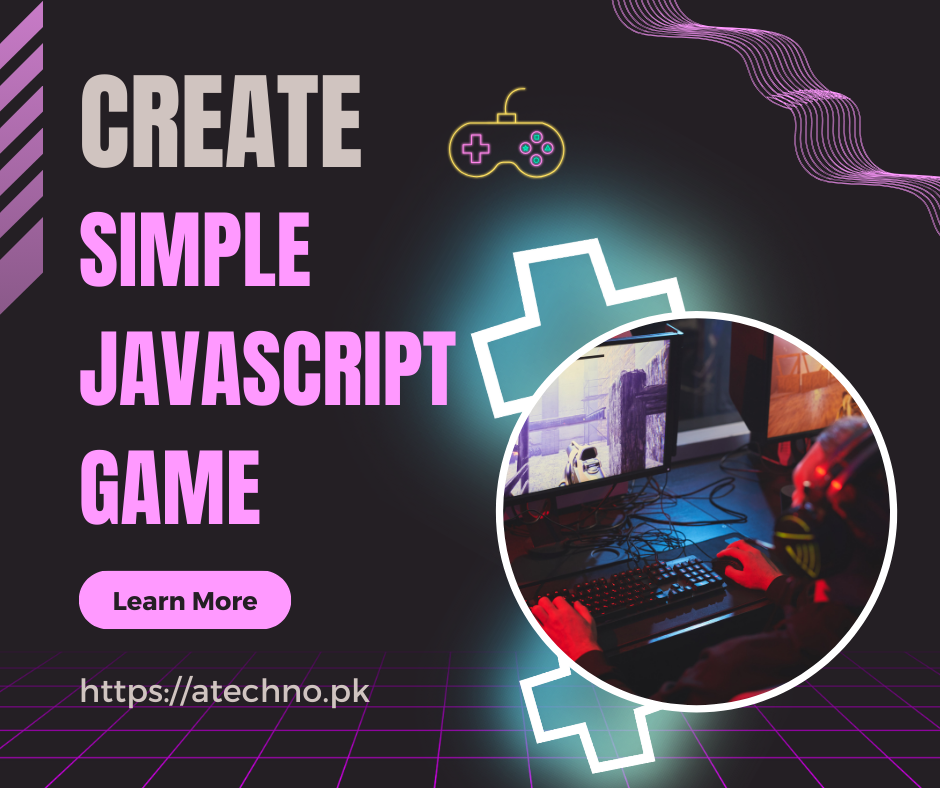
In the vast world of web development, JavaScript stands out as a versatile language that not only powers the interactive elements on websites but can also be used to create simple games in JavaScript. If you’re a coding enthusiast or a budding developer looking to dip your toes into game development, this article will guide you through the process of creating a basic game using JavaScript.
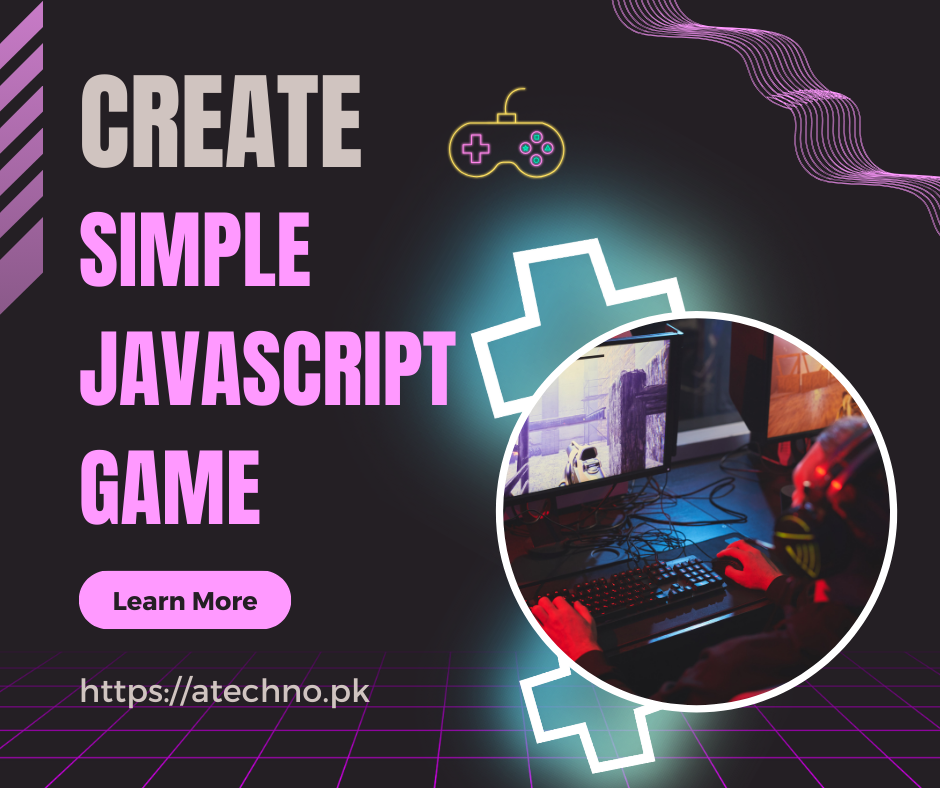
Table of Content
- Understanding the Basics
- Keep It Simple
- Creating the Canvas
- Making It Visually Appealing
- Bringing Your Game to Life
- Interactivity Matters
- The Brain Behind the Scenes
- Perfecting Your Creation
- Enhancing User Engagement
- It’s All in the Details
Understanding the Basics
Before diving into game development, ensure you have a solid understanding of HTML, CSS, and JavaScript. Familiarize yourself with the Document Object Model (DOM) as it plays a crucial role in creating interactive elements on a webpage.
Keep It Simple
Choosing the right game concept is essential, especially for beginners. Start with a simple game idea to grasp the fundamentals. Consider classics like “Guess the Number” or “Memory Game” as your initial projects.
Creating the Canvas
Begin by setting up the HTML structure. Create a canvas element, the virtual space where your game will unfold. Utilize the <canvas> tag and set its width and height attributes to define the game area.
Making It Visually Appealing
Although the focus is on JavaScript, a visually appealing game enhances the overall experience. Use CSS to style your canvas, providing a pleasant backdrop for your game. Apply basic styling to elements, ensuring a neat and organized layout.
Bringing Your Game to Life
The core of game development lies in JavaScript. Write functions to handle user input, manage game logic, and update the game state. Implement event listeners to capture user actions and manipulate the DOM to reflect changes in real-time.
Interactivity Matters
For many games, user input is a critical element. Implement functions to capture key presses or mouse clicks, allowing players to interact with your game. Use conditional statements to respond to different inputs and trigger corresponding actions.
The Brain Behind the Scenes
Define the rules and mechanics of your game through JavaScript functions. Whether it’s checking for a correct guess or updating the player’s score, this is where the magic happens. Break down complex logic into manageable steps for better understanding and debugging.
Perfecting Your Creation
As you progress, regularly test your game to identify and fix bugs. The browser’s developer tools will become your best friend during this phase. Console.log statements can help trace the flow of your code and pinpoint any issues.
Enhancing User Engagement
Elevate your game by incorporating simple animations. Utilize JavaScript’s built-in functions or explore libraries like Anime.js for more advanced animations. Smooth transitions and visual effects can significantly enhance the overall gaming experience.
It’s All in the Details
Pay attention to the user interface (UI) to ensure a seamless experience. Add informative messages, update scores dynamically, and provide visual feedback for user actions. A well-crafted UI contributes to the overall professionalism of your game.
Create A Simple JAVASCRIPT Game
Create a Simple Index.html file and add this code to your HTML file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>Guess the Number Game</title>
</head>
<body>
<div class="container">
<h1>Guess the Number Game</h1>
<p>Guess a number between 1 and 100:</p>
<input type="number" id="userGuess" placeholder="Enter your guess">
<button onclick="checkGuess()">Submit Guess</button>
<p id="message"></p>
</div>
<script src="script.js"></script>
</body>
</html>
Now Create a CSS File and name your CSS file to Style.css
body {
font-family: 'Arial', sans-serif;
background-color: #f5f5f5;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
.container {
text-align: center;
background-color: #fff;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
button {
padding: 10px;
margin-top: 10px;
cursor: pointer;
}
input, button {
font-size: 16px;
}
Now create your JS file and name your JS file Script.js and the following js code in the file:
// Generate a random number between 1 and 100
const secretNumber = Math.floor(Math.random() * 100) + 1;
// Get elements from the DOM
const userGuessInput = document.getElementById('userGuess');
const messageElement = document.getElementById('message');
// Function to check the user's guess
function checkGuess() {
// Get the user's guess
const userGuess = parseInt(userGuessInput.value);
// Check if the guess is correct
if (userGuess === secretNumber) {
showMessage('Congratulations! You guessed the correct number.', 'green');
} else {
showMessage('Wrong guess. Try again!', 'red');
}
}
// Function to display messages
function showMessage(message, color) {
messageElement.textContent = message;
messageElement.style.color = color;
}
Conclusion:
Creating a simple game in JavaScript is an exciting and rewarding endeavor for anyone interested in web development. By following these steps, you’ll gain hands-on experience, honing your coding skills while unleashing your creativity. Remember, practice makes perfect, so don’t hesitate to experiment and explore more complex game ideas as you become more proficient in JavaScript game development. Happy coding!
Post a Comment